Mu0n
Well-known member
Let's get what I'm using as gear out of the way:
Dev Machine:
modern PC with Basilisk II set with System 7.5.5, Symantec C++ 6.0
Target Machine:
SE/30 with System 7.5.3, no Apple MIDI Manager (prefer not to use it since I want to target 6.0.8 and a Mac Plus as well)
PocketMac MIDI interface plugged in the modem port, has 2 MIDI OUT (only using 1) 1 MIDI IN (not using it...for now)
Roland SC-88ST to accept midi signals from the mac and play stuff
Goals:
1) at least play a scale on channel #0, in sync with NoteOn and NoteOff effects
2) load a .mid file and play it using a fine-grain enough delay function as part of a game title screen at first - I'd adapt a python project I have that already works on a modern PC.
3) same as 2, but use interrupt+callback functions to allow game logic, graphics, inputs, not sure how far I can push the old 68k macs (it was painful in Space Quest III on the Mac Plus but there was a lot going with their interpreter as well)
Progress made:
I've already made tons of tests with some python code running on my PC, I can parse a .mid file and deal with the 2 main cases of a type 0 .mid file (every data stuck in 1 track) and type 1 .mid file (info separated into a bunch of tracks, typically 4-8, but can go up to 22 sometimes) and using a delay function to sit idle and respect the events' time delta-to-go information. If you're curious, my code sits at: https://github.com/Mu0n/PythonMidiPlayer
I've settled on using documentation from here:
Inside Macintosh: Serial Driver
Needs:
I need the extra step to make it MIDI compliant and well synced.
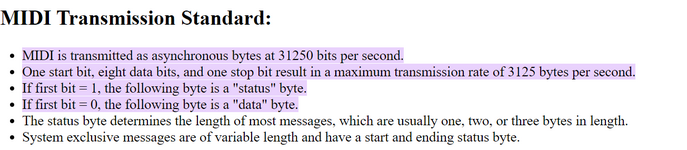
But, SerRest, PBWrite, OpenDriver and such from the inside mac reference above allow me these settings:
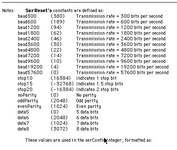
The first configuration I tried was the default one from the documentation:
9600 bps + 1 stop bit + no parity + 8 data bits and I was met with complete silence.
2nd attempt was to use 19200 bps, and I try to send the note event 5 times.
Only the first and last event do something, there's a huge sync problem.
(put your sound way up):
3rd attempt was to use 57600 and that's total silence again.
How does stuff like cubase and other sequencers do it? They let you pick the modem or serial port and let you choose the base transmission speed like 0.5 MHz, 1 MHz, etc.
I notice that 1 000 000 MHz / 32 is exactly what MIDI wants, 31250.
Help!
Dev Machine:
modern PC with Basilisk II set with System 7.5.5, Symantec C++ 6.0
Target Machine:
SE/30 with System 7.5.3, no Apple MIDI Manager (prefer not to use it since I want to target 6.0.8 and a Mac Plus as well)
PocketMac MIDI interface plugged in the modem port, has 2 MIDI OUT (only using 1) 1 MIDI IN (not using it...for now)
Roland SC-88ST to accept midi signals from the mac and play stuff
Goals:
1) at least play a scale on channel #0, in sync with NoteOn and NoteOff effects
2) load a .mid file and play it using a fine-grain enough delay function as part of a game title screen at first - I'd adapt a python project I have that already works on a modern PC.
3) same as 2, but use interrupt+callback functions to allow game logic, graphics, inputs, not sure how far I can push the old 68k macs (it was painful in Space Quest III on the Mac Plus but there was a lot going with their interpreter as well)
Progress made:
I've already made tons of tests with some python code running on my PC, I can parse a .mid file and deal with the 2 main cases of a type 0 .mid file (every data stuck in 1 track) and type 1 .mid file (info separated into a bunch of tracks, typically 4-8, but can go up to 22 sometimes) and using a delay function to sit idle and respect the events' time delta-to-go information. If you're curious, my code sits at: https://github.com/Mu0n/PythonMidiPlayer
I've settled on using documentation from here:
Inside Macintosh: Serial Driver
Needs:
I need the extra step to make it MIDI compliant and well synced.
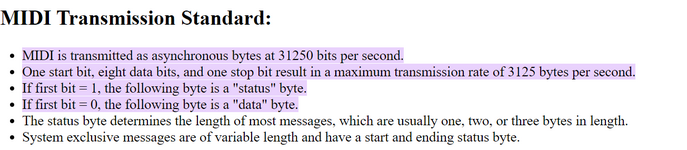
But, SerRest, PBWrite, OpenDriver and such from the inside mac reference above allow me these settings:
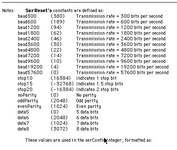
The first configuration I tried was the default one from the documentation:
9600 bps + 1 stop bit + no parity + 8 data bits and I was met with complete silence.
2nd attempt was to use 19200 bps, and I try to send the note event 5 times.
Only the first and last event do something, there's a huge sync problem.
(put your sound way up):
3rd attempt was to use 57600 and that's total silence again.
How does stuff like cubase and other sequencers do it? They let you pick the modem or serial port and let you choose the base transmission speed like 0.5 MHz, 1 MHz, etc.
I notice that 1 000 000 MHz / 32 is exactly what MIDI wants, 31250.
Help!