jkheiser
Well-known member
I started tinkering on this a month ago and thought I would start sharing my progress about it here on 68kMLA.
Why assembly? Good question. The short answer: it’s just a self-imposed challenge. The long answer: I grew up using a Mac Plus, which gave me the skills that led to an enjoyable career in computers. Despite this, I was never more than a power user. Other than World Builder and BASIC, I avoided programming because it intimidated me. I later overcame this aversion and now enjoy writing JavaScript for a living. Even so, part of me is still afraid of “real programming,” the kind where you’re actually writing instructions for the processor to push bytes around. It’s always looked like an impenetrable soup of hexadecimal gibberish I could never understand, but to hell with that: I’m going to learn 68k assembly and make a fun game before I turn 50 next year.
Other goals for this project:
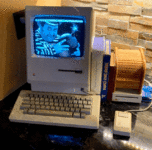
Why assembly? Good question. The short answer: it’s just a self-imposed challenge. The long answer: I grew up using a Mac Plus, which gave me the skills that led to an enjoyable career in computers. Despite this, I was never more than a power user. Other than World Builder and BASIC, I avoided programming because it intimidated me. I later overcame this aversion and now enjoy writing JavaScript for a living. Even so, part of me is still afraid of “real programming,” the kind where you’re actually writing instructions for the processor to push bytes around. It’s always looked like an impenetrable soup of hexadecimal gibberish I could never understand, but to hell with that: I’m going to learn 68k assembly and make a fun game before I turn 50 next year.
Other goals for this project:
- Good-quality sampled sound effects (11KHz) using the original sound driver
- Good-quality animation (at least 20fps) using CopyBits
- Compatible with early Macs, even the original 128k
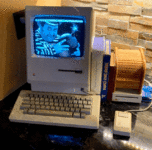